Organize your Arduino code with header and class files
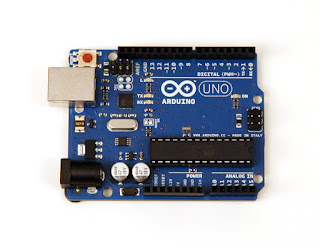
Download Example Code Here Ok, so you made your awesome Arduino widget, but now you're looking at the code trying to find the spot where you made it do that cool thing, and you can't find it! There's just too much going on in the sketch file! Well my friend, it might be time for you to start organizing your code using classes and class files. What are C++ classes? A class is basically a description of the variables and actions that something in your application might be interested in. For instance, if you were making a application involving vehicles, you might make a class that holds the speed the vehicle is currently traveling, and might have actions like drive and stop. Something like this: class vehicle{ private: int CurrentSpeed; public: void drive(int speed){ //increase the speed CurrentSpeed = speed; } void stop(){ // decrease the speed while(CurrentSpeed>0){ CurrentSpeed--; } }; The clas