Using GIT with Arduino
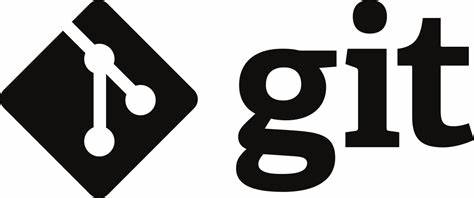
What is GIT? The term GIT doesn't actually mean anything. It was made up. But what it is, is a "version control system". That sounds complicated, but really all it boils down to is that it saves versions of your files, and gives you a way to revert back to old versions - if necessary. It also provides a way for multiple developers to work on the same project, and even to keep the same project 'in sync' between computers no matter where they are. Where do I get it? In order to use GIT, you need to install it. Many IDE's come with it pre-installed, but unfortunately, the Arduino IDE does not. You can find downloads for Windows, Mac, or Linux here: https://git-scm.com/downloads I will note, however, that GIT is generally a Command-Line tool. There are GUI's also available on the same website. If you need a GUI, then you can get one there. You should first learn the basic commands for GIT before using a UI though. Once you download and install it, you can...